THIS TUTORIAL IS OUTDATED. DO NOT USE. AN ALTERNATIVE CAN BE FOUND HERE
Hey there,
since coolade's tutorial on addon coding is now outdated, I'll try to write a new one. We'll be making the same ability "Ignite".
Let's begin!
(I am in no way stealing Coolade's code)
Step 1
If you don't have any experience with Java coding, you'll have to learn that first. Google and YouTube are your friends. Also, this site might help.
Now, if you don't have any experience with bukkit, you'll have to work on that first as well. Here are some videos on bukkit/spigot coding. This channel has more videos, so you can check out more of them. Make sure that you get to listeners at least.
These are general things that are useful for this. Also, make sure you have spigot. You can find it at www.spigotmc.org.
Step 3
Now, we're starting our ability. Just like Coolade's tutorial, we'll be making an ability called ignite. Download the latest ProjectKorra build (The one your server uses at least) and you're ready to start!
Open Eclipse
Create a new project
Name it Ignite. Then hit enter.
Right click the project, and click 'Properties'.
Then under 'Resource', go to 'Java Build Path', then click 'Libraries'. Then add both spigot and ProjectKorra.
Good job, you have created the project! Now, let's get into creating the files and code.
Step 4
Right click the ignite project, and create a new package. (new > package)
Name the package "me.insert name here.korra.ignite", so in my case, "me.finnbueno.korra.ignite".
Right click the package, and create a new class. (new > class)
We're going to do this 2 times.
Name the first class 'IgniteInformation'.
Name the second class 'IgniteListener'.
Then, right click the project again (Not the package!). Create a new file now. (new > file)
Name it 'path.yml'. Click finish.
Now you should have 2 classes, named 'IgniteInformation' and 'IgniteListener'. You should also have a file called 'path.yml'. If so, good job. Now we'll start the real coding, I promise!
Step 5
First, we'll be doing the IgnoreInformation class.
This class will contain all the code needed to register the ability.
First, extend the class 'AbilityModule':
Import AbilityModule. Now there should be a red line under IngiteInformation. To fix this, we'll have to add a constructor, which calls the super constructor with the name of the ability:
Now, IgniteInformation should still give an error. That's because there are more methods needed. Hover over IgniteInformation and clicked, "Add unimplented methods." Then, you should get this:
I'll try to go over each method as detailed as possible.
the author method:
This tells the plugin who made the ability. Mainly used for debugging. Replace null with a string containing your name. In my case, "Finn_Bueno_" (Including the brackets of course!).
The description method:
This is shown when the player types /bending help ignite. It automatically gets the element's colour, so you dont have to worry about that. For this ability, replace null with:
The element method:
This method tells the plugin for what element the ability is. Note that it wants a string, so we'll have to covert the element to a string. (Or just type "Fire"). Replace null with Element.Fire.toString();
The version method:
This method isn't too important. It simply gets the version of the ability. Mainly for debugging. Replace null with "1.0V" (Including brackets!)
The harmless and shift method:
They are pretty self-explanatory. If isHarmlessAbility is set to true, players will be able to use this in protected areas. (If set so in the config.). If isSneakAbility is set to true, people will be able to use swiftswim or other passives like that while on that ability.
The onThisLoad method:
In my opinion, the most important of all. The code in this method will be called when the ability loads. In this method, we'll register our listener class, add a permission, and set the permission default.
First, add this in the onThisLoad method.
Since we're not coding a plugin, we'll assign it to ProjectKorra.
This tells spigot/the server/idk that we want to listen for events in IgniteListener.
Now, to add the permission, type the following.
This adds a new permission, "bending.ability.Ignite". Adding this to a player or group will give them access to the ability.
Now, this is a basic ability so we want players to be allowed to use it by default. To do this, type the following.
If you still want a group or player to not have access to the ability, add "-bending.ability.Ignite" to them.
That's it for the onThisLoad().
IgniteListener will be continued in the next post. (10000 character cap)
Hey there,
since coolade's tutorial on addon coding is now outdated, I'll try to write a new one. We'll be making the same ability "Ignite".
Let's begin!
(I am in no way stealing Coolade's code)
Step 1
If you don't have any experience with Java coding, you'll have to learn that first. Google and YouTube are your friends. Also, this site might help.
Step 2
Now, if you don't have any experience with bukkit, you'll have to work on that first as well. Here are some videos on bukkit/spigot coding. This channel has more videos, so you can check out more of them. Make sure that you get to listeners at least.
These are general things that are useful for this. Also, make sure you have spigot. You can find it at www.spigotmc.org.
Step 3
Now, we're starting our ability. Just like Coolade's tutorial, we'll be making an ability called ignite. Download the latest ProjectKorra build (The one your server uses at least) and you're ready to start!
Open Eclipse
Create a new project
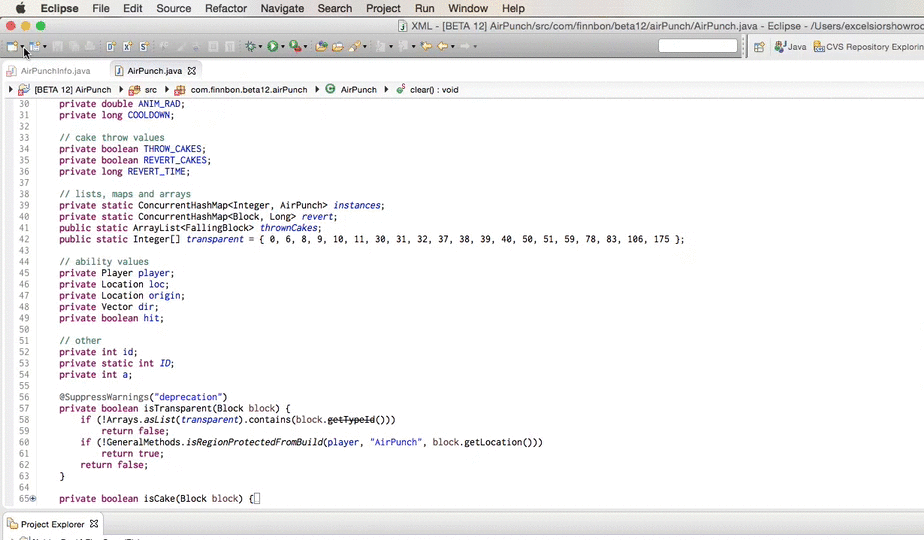
Right click the project, and click 'Properties'.
Then under 'Resource', go to 'Java Build Path', then click 'Libraries'. Then add both spigot and ProjectKorra.
Good job, you have created the project! Now, let's get into creating the files and code.
Step 4
Right click the ignite project, and create a new package. (new > package)
Name the package "me.insert name here.korra.ignite", so in my case, "me.finnbueno.korra.ignite".
Right click the package, and create a new class. (new > class)
We're going to do this 2 times.
Name the first class 'IgniteInformation'.
Name the second class 'IgniteListener'.
Then, right click the project again (Not the package!). Create a new file now. (new > file)
Name it 'path.yml'. Click finish.
Now you should have 2 classes, named 'IgniteInformation' and 'IgniteListener'. You should also have a file called 'path.yml'. If so, good job. Now we'll start the real coding, I promise!
Step 5
First, we'll be doing the IgnoreInformation class.
This class will contain all the code needed to register the ability.
First, extend the class 'AbilityModule':
Code:
public class IngiteInformation extends AbilityModule {
}
Code:
public class IngiteInformation extends AbilityModule {
public IgniteInformation() {
super("Ignite");
}
}
Code:
public class IgniteInformation extends AbilityModule {
public IngiteInformation() {
super("Ignite");
}
@Override
public String getAuthor() {
// TODO Auto-generated method stub
return null;
}
@Override
public String getDescription() {
// TODO Auto-generated method stub
return null;
}
@Override
public String getElement() {
// TODO Auto-generated method stub
return null;
}
@Override
public String getVersion() {
// TODO Auto-generated method stub
return null;
}
@Override
public boolean isHarmlessAbility() {
// TODO Auto-generated method stub
return false;
}
@Override
public boolean isShiftAbility() {
// TODO Auto-generated method stub
return false;
}
@Override
public void onThisLoad() {
}
}
the author method:
Code:
@Override
public String getAuthor() {
// TODO Auto-generated method stub
return null;
}
The description method:
Code:
@Override
public String getDescription() {
// TODO Auto-generated method stub
return null;
}
(Again, including brackets!)"This ability allows a firebender to set someone on fire by simply punching them!"
The element method:
Code:
@Override
public String getElement() {
// TODO Auto-generated method stub
return null;
}
The version method:
Code:
@Override
public String getVersion() {
// TODO Auto-generated method stub
return null;
}
The harmless and shift method:
Code:
@Override
public boolean isHarmlessAbility() {
// TODO Auto-generated method stub
return false;
}
@Override
public boolean isShiftAbility() {
// TODO Auto-generated method stub
return false;
}
The onThisLoad method:
Code:
@Override
public void isHarmlessAbility() {
// TODO Auto-generated method stub
}
First, add this in the onThisLoad method.
Code:
ProjectKorra.plugin.getServer().getPluginManager().registerEvents(new IgniteListener(), ProjectKorra.plugin);
This tells spigot/the server/idk that we want to listen for events in IgniteListener.
Now, to add the permission, type the following.
Code:
ProjectKorra.plugin.getServer().getPluginManager().addPermission(new Permission("bending.ability.Ignite"));
Now, this is a basic ability so we want players to be allowed to use it by default. To do this, type the following.
Code:
ProjectKorra.plugin.getServer().getPluginManager().getPermission("bending.ability.Ignite").setDefault(PermissionDefault.TRUE);
That's it for the onThisLoad().
IgniteListener will be continued in the next post. (10000 character cap)
Last edited: